A tutorial is now available on Code Project, so check the article for a detailed overview. And please leave your rating if you like the control 🙂
http://www.codeproject.com/KB/WPF/versatile_treeview.aspx
Update: The latest version is currently only available through the download link below. I’ll update the CodeProject article once the current filtering mechanism has been rewritten:
Download: wpf-treeview.zip (Current version: 1.0.7, updated 2008.04.06)
This is a little something I’ve been working on for a while: A replacement (or better: enhancement) of WPF’s built-in TreeView control.
I became aware of the default control’s limitations during my last project – I naturally started with hierarchical data templates, but was soon confronted with quite a few issues: I missed a simple API to control the tree, and styling of the tree’s nodes proved hard as well. Furthermore, WPF’s TreeView tends to fire all sorts of SelectedItemChanged events if it’s being refreshed or rebound, which caused side-effects with TwoWay data binding.
However, instead of posting a rant that probably nobody would ever read (let alone care about), I worked on an alternative. Here’s the tree’s main features at a glance:
- Simple declaration:
<local:ProductTree x:Name="MyTree"
Items="{Binding Source={StaticResource Shop},
Path=Products}"
SelectedItem="{Binding ElementName=MyProductList,
Path=ActiveItem, Mode=TwoWay}"
NodeContextMenu="{StaticResource CategoryMenu}"
TreeNodeStyle="{StaticResource SimpleFolders}"
TreeStyle="{StaticResource SimpleTreeStyle}"
SelectedItemChanged="OnSelectedItemChanged"
/>
All this goodness comes at a price: The TreeViewBase class that provides this functionality, is abstract. This means you have to write a little code yourself. However, you’ll probably manage with 3 lines of code, as the base control just needs to know 3 things:
- How to generate an identifier for a given tree node
- How to access a bound item’s childs, if there are any
- How to access a bound item’s parent, if there is one
Here’s the complete implementation of the sample application’s tree control:
//a tree control that handles only ShopCategory objects
public class CategoryTree : TreeViewBase<ShopCategory>
{
//the sample uses the category's name as the identifier
public override string GetItemKey(ShopCategory item)
{
return item.CategoryName;
}
//returns subcategories that should be available through the tree
public override ICollection<ShopCategory>
GetChildItems(ShopCategory parent)
{
return parent.SubCategories;
}
//get the parent category, or null if it's a root category
public override ShopCategory GetParentItem(ShopCategory item)
{
return item.ParentCategory;
}
I’m planning to write a CodeProject article for this one, but for now, it’s only available through my place without a tutorial. However: The library comes with a sample project that shows pretty much all features of the control. Project format is currently VS2008 only, but binaries which target .NET 3.0 are included. Enjoy!
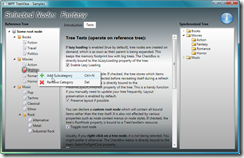